Test Drive: Qwen2.5 + Openrouter + LiteLLM
Published on .
Today I’m testing driving the new Qwen2.5 model through OpenRouter via the LiteLLM Python SDK. I’m going to make an ultra simple car listings Django project since Qwen2.5 purports to have greatly improved coding skills. The process involves:
- Get an OpenRouter key
- Engineer a prompt
- Invoke Qwen
After setting things up and paying $5 at https://openrouter.ai/credits I used the following prompt. I followed the instructions exactly and got a working Django app with seed data. Wowzaz! So far I’ve discovered one easily resolvable reverse()
error.
from litellm import completion
from textwrap import dedent
response = completion(
model="openrouter/qwen/qwen-2.5-72b-instruct",
messages=[{ "content": dedent("""\
Architect a django project with an app for car listings.
Include models for:
- Listings
Include views for:
- see all listings
- see a single listing
- add and modify a listing
Include a seeder for listings"""), "role": "user"}],
)
print(response.choices[0].message.content)
It produced the app in the screenshots below:
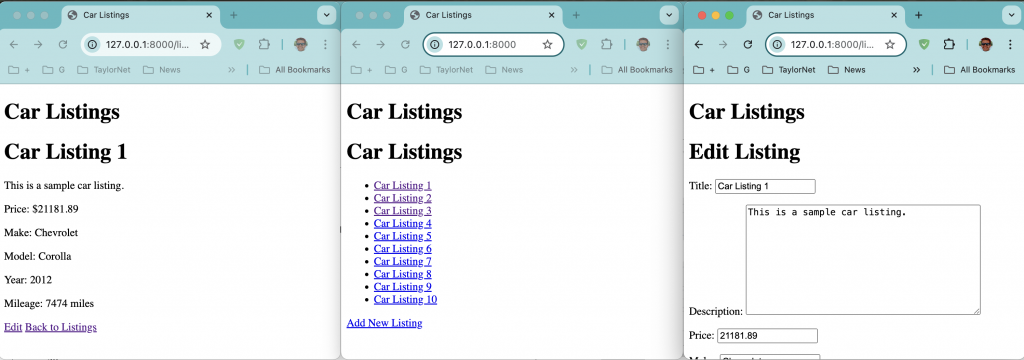
Here’s the Markdown it returned.
Certainly! Below is a step-by-step guide to creating a Django project with an app for car listings. This guide includes models, views, and a seeder for the listings.
Step 1: Set Up the Django Project
Create a new Django project:
django-admin startproject carlistings
cd carlistingsCreate a new app called
listings
:python manage.py startapp listings
Add the
listings
app toINSTALLED_APPS
insettings.py
:INSTALLED_APPS = [
...
'listings',
]
Step 2: Define the Models
Create the
Listing
model inlistings/models.py
:from django.db import models
class Listing(models.Model):
title = models.CharField(max_length=255)
description = models.TextField()
price = models.DecimalField(max_digits=10, decimal_places=2)
make = models.CharField(max_length=100)
model = models.CharField(max_length=100)
year = models.IntegerField()
mileage = models.IntegerField()
created_at = models.DateTimeField(auto_now_add=True)
updated_at = models.DateTimeField(auto_now=True)
def __str__(self):
return self.titleApply the migrations:
python manage.py makemigrations
python manage.py migrate
Step 3: Create Views
Create the views in
listings/views.py
:from django.shortcuts import render, get_object_or_404, redirect
from .models import Listing
from .forms import ListingForm
def list_listings(request):
listings = Listing.objects.all()
return render(request, 'listings/list_listings.html', {'listings': listings})
def view_listing(request, listing_id):
listing = get_object_or_404(Listing, pk=listing_id)
return render(request, 'listings/view_listing.html', {'listing': listing})
def add_listing(request):
if request.method == 'POST':
form = ListingForm(request.POST)
if form.is_valid():
form.save()
return redirect('list_listings')
else:
form = ListingForm()
return render(request, 'listings/add_listing.html', {'form': form})
def modify_listing(request, listing_id):
listing = get_object_or_404(Listing, pk=listing_id)
if request.method == 'POST':
form = ListingForm(request.POST, instance=listing)
if form.is_valid():
form.save()
return redirect('view_listing', listing_id=listing.id)
else:
form = ListingForm(instance=listing)
return render(request, 'listings/modify_listing.html', {'form': form})Create the form in
listings/forms.py
:from django import forms
from .models import Listing
class ListingForm(forms.ModelForm):
class Meta:
model = Listing
fields = ['title', 'description', 'price', 'make', 'model', 'year', 'mileage']
Step 4: Create Templates
Create the directory structure for
templates:mkdir -p listings/templates/listings
Create the templates:
listings/templates/listings/list_listings.html
:{% extends 'base.html' %}
{% block content %}
<h1>Car Listings</h1>
<ul>
{% for listing in listings %}
<li>
<a href="{% url 'view_listing' listing.id %}">{{ listing.title }}</a>
</li>
{% endfor %}
</ul>
<a href="{% url 'add_listing' %}">Add New Listing</a>
{% endblock %}listings/templates/listings/view_listing.html
:{% extends 'base.html' %}
{% block content %}
<h1>{{ listing.title }}</h1>
<p>{{ listing.description }}</p>
<p>Price: ${{ listing.price }}</p>
<p>Make: {{ listing.make }}</p>
<p>Model: {{ listing.model }}</p>
<p>Year: {{ listing.year }}</p>
<p>Mileage: {{ listing.mileage }} miles</p>
<a href="{% url 'modify_listing' listing.id %}">Edit</a>
<a href="{% url 'list_listings' %}">Back to Listings</a>
{% endblock %}listings/templates/listings/add_listing.html
:{% extends 'base.html' %}
{% block content %}
<h1>Add New Listing</h1>
<form method="post">
{% csrf_token %}
{{ form.as_p }}
<button type="submit">Save</button>
</form>
<a href="{% url 'list_listings' %}">Back to Listings</a>
{% endblock %}listings/templates/listings/modify_listing.html
:{% extends 'base.html' %}
{% block content %}
<h1>Edit Listing</h1>
<form method="post">
{% csrf_token %}
{{ form.as_p }}
<button type="submit">Save</button>
</form>
<a href="{% url 'view_listing' listing.id %}">Back to Listing</a>
<a href="{% url 'list_listings' %}">Back to Listings</a>
{% endblock %}
Create a base template
listings/templates/base.html
:<!DOCTYPE html>
<html>
<head>
<title>Car Listings</title>
</head>
<body>
<header>
<h1>Car Listings</h1>
</header>
<main>
{% block content %}{% endblock %}
</main>
</body>
</html>
Step 5: Define URLs
Create
listings/urls.py
:from django.urls import path
from . import views
urlpatterns = [
path('', views.list_listings, name='list_listings'),
path('listings/<int:listing_id>/', views.view_listing, name='view_listing'),
path('listings/add/', views.add_listing, name='add_listing'),
path('listings/<int:listing_id>/edit/', views.modify_listing, name='modify_listing'),
]Include the app URLs in the project’s
urls.py
:from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('listings.urls')),
]
Step 6: Create a Seeder
Create a seeder script in
listings/management/commands/seed_listings.py
:from django.core.management.base import BaseCommand
from listings.models import Listing
import random
class Command(BaseCommand):
help = 'Seed the database with sample car listings'
def handle(self, *args, **options):
makes = ['Toyota', 'Honda', 'Ford', 'Chevrolet', 'Nissan']
models = ['Corolla', 'Civic', 'F-150', 'Silverado', 'Altima']
for i in range(10):
listing = Listing(
title=f'Car Listing {i + 1}',
description='This is a sample car listing.',
price=round(random.uniform(5000, 50000), 2),
make=random.choice(makes),
model=random.choice(models),
year=random.randint(2000, 2023),
mileage=random.randint(1000, 200000)
)
listing.save()
self.stdout.write(self.style.SUCCESS('Successfully seeded the database with 10 listings'))Run the seeder:
python manage.py seed_listings
Step 7: Run the Development Server
Run the development server:
python manage.py runserver
Visit
http://127.0.0.1:8000/
in your web
browser to see the listings.
That’s it! You now have a Django project with an app for car listings, including models, views, and a seeder.